This article is a guide to the Array data structure in Godot. It is written for beginner and expert developers using the Godot engine. As you will see, an array is a very robust data structure that you can use for your needs. Treat this article as a cheat sheet for all array functions in Godot.
What Is an Array in Godot?
An array in Godot is a list of elements stored next to each other in memory, whether the elements are simple types like integers or complex objects like resources or nodes. Arrays can store any kind of element, even mixing types in a single array.
You can perform many operations on arrays, such as adding or removing elements, reading and writing existing elements, and manipulating the values in the array, like reversing or shuffling the array order, among many others.
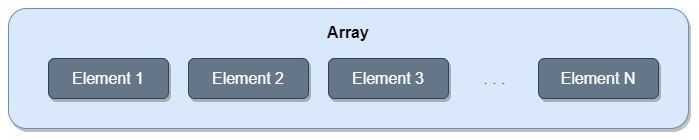
Why Do I Need to Use Arrays?
Arrays are a fundamental building block of every software. As fun as it is to declare individual variables, it is sometimes impossible to access and manage a large amount of them. Arrays are one of the best data structures for automating your game systems, as they give you the ability to store and access large amounts of data sequentially with a small amount of code.
Here are some examples of arrays:
- An array of current volume levels for each audio bus, stored as integers or floats.
- An array of scene names or paths, stored as strings, managed by a Scene Manager.
- An array of enemy objects and all their properties, stored as custom resources.
Note: In many cases, using arrays increases the game’s performance since all data in an array is found in a contiguous chunk of memory. This is not always the case, but it’s worth remembering, especially if you work with a large amount of stored information.
How to Declare an Array in Godot?
There are two ways to define an array in Godot in terms of typing: typed arrays and non-typed arrays.
Typed arrays prevent you or any other developers you work with from accidentally adding elements to the array that have the wrong type. This is great for avoiding bugs during development but limits the array to a specific type of variable.
var typedArray : Array[String]
On the other hand, you can define a non-typed array, which can store any kind of variable or even mixed kinds. This gives you flexibility in storage and access to the data but opens up the potential for bugs later on.
var nonTypedArray : Array
How to Declare an Array With Predefined Values?
Sometimes, you might want to create an array and set a list of values to it right away.
var predefinedArray : Array[String] = ["Sword", "Shield", "Helmet"]
This technique doesn’t have to be in the array declaration. You can do this in any part of your code. Initialize the array with the empty list symbol []
, and assign the values later in code.
var anArray : Array[String] = []
anArray = ["Sword", "Shield", "Helmet"]
How to Resize an Array to a Specific Size?
Arrays don’t have to start with zero elements, and you don’t have to add or remove elements to change their size. You can instruct Godot to resize an array to the specific number of elements you provide. Keep in mind that all created elements, for non-typed arrays, will initially have a value of null
. For typed arrays, the new elements will have the default value of the assigned type.
var anArray : Array[String] = ["Sword", "Shield", "Helmet"]
var arraySize : int = 8
anArray.resize(arraySize)
print(anArray) # Result: ["Sword", "Shield", "Helmet", "", "", "", "", ""]
How to Fill an Array With Specific Values?
In some cases, you might want to fill an array with a predefined value so that all elements have that specific value you provide. This is useful for initializing an array, among other situations. For example, resizing an array to a known size and filling it with zeros is a good way to initialize an array.
var anArray : Array[int] = []
var arraySize : int = 8
anArray.resize(arraySize)
anArray.fill(0)
print(anArray) # Result: [0, 0, 0, 0, 0, 0, 0, 0]
How to Access and Modify an Array in Godot?
Arrays excel at storing and accessing data from memory. Godot allows you to access the array by performing basic data operations such as adding, removing, reading, or writing elements from the array’s memory.
How to Add Elements to an Array in Godot?
Adding new elements to an array is relatively simple. There are two main use cases for adding elements: appending data at the end of the array, and inserting data in the beginning or middle of the array. In most cases, you would want to append data at the end of the array. However, in some situations, you might also need to insert elements in the middle of the array. In both cases, adding a new element will increase the size of the array by one.
var anArray : Array[String] = ["Sword", "Shield", "Helmet"]
anArray.append("Boots")
print(anArray) # Result: ["Sword", "Shield", "Helmet", "Boots"]
How to Insert Elements in the Middle of an Array in Godot?
When inserting a new element into an array, you need to provide the index where you want your element to be inserted. Note that elements that come after the given index will be shifted one index up.
var anArray : Array[String] = ["Sword", "Shield", "Helmet"]
anArray.insert(1, "Boots")
print(anArray) # Result: ["Sword", "Boots", "Shield", "Helmet"]
How to Remove an Element From an Array?
There are two ways to remove an element from an array:
- By Index: The element at the given index is erased from the array. All other elements shift down by one index.
- By Value: The first element with the given value is erased from the array. If there are more elements with the same value, they will remain in the array. All other elements shift down by one index.
var array1 : Array[String] = ["Sword", "Shield", "Helmet", "Boots"]
# Remove item by value
array1.erase("Shield")
print(array1) # Result: ["Sword", "Helmet", "Boots"]
# Remove item by index
var array2 : Array[String] = ["Sword", "Shield", "Helmet", "Boots"]
array2.remove_at(1)
print(array2) # Result: ["Sword", "Helmet", "Boots"]
How to Write a Value to an Element in an Array?
To write a value, use the extraction operator []
and specify the index you want to change. Then, assign a new value to it with the assignment operator.
var anArray : Array[String] = ["Sword", "Shield", "Helmet"]
anArray[1] = "Boots"
print(anArray) # Result: ["Sword", "Boots", "Helmet"]
How to Read an Element Value in an Array?
To read a value, use the extraction operator []
and specify the index you want to read.
var anArray : Array[String] = ["Sword", "Shield", "Helmet"]
print(anArray[1]) # Result: "Shield"
How to Get the Size of an Array in Godot?
The size of an array is equal to the number of elements found in that array. Keep in mind that the returned size is the actual number of items in the array, while the indices range from 0 to (size – 1).
var anArray : Array[int] = [1, 2, 4, 8]
print(anArray.size()) # Result: 4
How to Go over an Array and Perform an Action on Each Item?
The easiest way to perform an action on each of the elements in an array is to use a for loop to iterate over all items and apply the action to each of them. For example, multiply all values in an array by 3.
var anArray : Array[int] = [1, 2, 4, 8]
for index in range(anArray.size()):
anArray[index] = anArray[index] * 3
print(anArray) # Result: [3, 6, 12, 24]
Warning: Be careful not to iterate over the array’s elements, as each element is the value itself and not the memory location.
var anArray : Array[int] = [1, 2, 4, 8]
for element in anArray:
element = element * 3 # Assigning a temporary variable
print(anArray) # Result: [1, 2, 4, 8]
How to Remove All Elements From an Array?
You can easily remove all elements from the array by assigning an empty array to it. If you have experience with low-level languages and you are worried about memory management, then don’t worry. Godot has got you covered with its internal garbage collector, which will release that memory.
var anArray : Array[int] = [1, 2, 4, 8]
anArray = [] # Clears the array
How to Reverse an Array in Godot?
Reversing an array is fairly straightforward. The only thing I would mention is that the operation is done in-place, meaning that the change is applied to the same array and you won’t get a new one instead.
var anArray : Array[int] = [1, 2, 4, 8]
anArray.reverse()
print(anArray) # Result: [8, 4, 2, 1]
How to Check if a Specific Value Exists in an Array?
In some instances, you might want to find out whether a specific value exists in an array. For example, checking if the player has a specific item in their inventory.
var inventory : Array[String] = ["Sword", "Shield", "Helmet"]
# Use the 'has' method to check if the value exists in the array
if (inventory.has("Shield")):
print("Shield is in the inventory")
else:
print("Shield is NOT in the inventory")
# Use the 'in' keyword to check if the value exists in the array
if ("Shield" in inventory):
print("Shield is in the inventory")
else:
print("Shield is NOT in the inventory")
How to Check if Two Arrays Are Equal in Godot?
Two arrays are considered equal if their sizes are equal and they have the exact same element values.
var array1 : Array[String] = ["Sword", "Shield", "Helmet"]
var array2 : Array[String] = ["Sword", "Shield", "Boots"]
if (array1 == array2): # Result: Arrays are NOT the same
print("Arrays are the same")
else:
print("Arrays are NOT the same")
How to Shuffle the Elements in an Array
Shuffling an array will cause the elements of the array to be reordered in a random manner. Result will probably be different each time you run the game. Similar to the reverse operation, the shuffle operation is done in-place as well.
var anArray : Array[String] = ["Sword", "Shield", "Helmet", "Boots"]
anArray.shuffle()
print(anArray) # Result: ["Boots", "Helmet", "Sword", "Shield"]
How to Find the Index of an Object in an Array?
Godot can find the index of a given element value. If the value is not found in the array, -1 will be returned.
var anArray : Array[String] = ["Sword", "Shield", "Helmet", "Boots"]
var helmetIndex : int = anArray.find("Helmet")
print(helmetIndex) # Result: 2
How to Combine Two Arrays Together
Combining two arrays together in Godot means that all elements in the second array will be appended at the end of the first array. A new array is returned as a result of this operation.
var array1 : Array[String] = ["Sword", "Shield"]
var array2 : Array[String] = ["Helmet", "Boots"]
var inventory : Array[String] = array1 + array2
print(inventory) # Result: ["Sword", "Shield", "Helmet", "Boots"]
How to Sort an Array in Ascending Order?
There is a special method for sorting arrays, simply called sort
. This method sorts the existing array in ascending order. Numerical values are sorted from low to high, and strings are sorted in alphabetical order.
var anArray : Array[int] = [4, 8, 1, 2]
anArray.sort()
print(anArray) # Result: [1, 2, 4, 8]
How to Duplicate an Array in Godot?
There are two levels of duplication in Godot: shallow copy and deep copy. Understanding the difference between them is crucial for avoiding incredibly difficult bugs later on.
Assigning a list to another list triggers a shallow copy of the array. A shallow-copied array will have two variables that point to the same area in memory. This means that modifying the array’s values through one variable will cause the other one to change as well, as they are pointing to the same area in memory.
var array1 : Array[int] = [1, 2, 4, 8]
var array2 : Array[int] = array1
array2[3] = 10
print(array1) # Result: [1, 2, 4, 10]
print(array2) # Result: [1, 2, 4, 10]
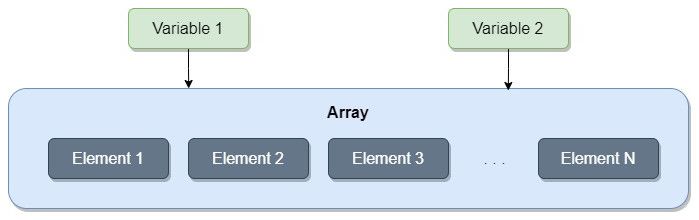
On the other hand, you can deep copy an array into a separate area of memory by actually duplicating all the data found in the array, which creates two distinct areas of memory with the same data. Now, when one of them is modified, the other one does not change. You do this by using the duplicate()
method.
var array1 : Array[int] = [1, 2, 4, 8]
var array2 : Array[int] = array1.duplicate()
array2[3] = 10
print(array1) # Result: [1, 2, 4, 8]
print(array2) # Result: [1, 2, 4, 10]
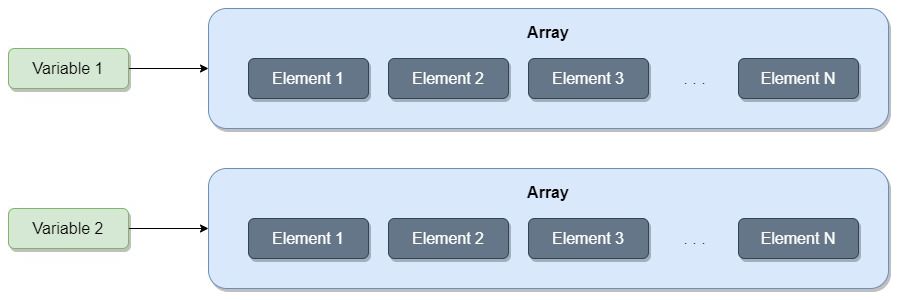
How to Find the Minimum and Maximum Values in an Array?
Godot provides built-in methods to find the numeric minimum and maximum in a given array. If the values can’t be compared, a null
value is returned.
var anArray : Array[int] = [4, 2, 8, 1]
print(anArray.min()) # Result: 1
print(anArray.max()) # Result: 8
How to Slice an Array and Get a Portion of It?
With slicing, you can extract a portion of the array into a new array. Going back to the inventory example, you can have a multi-page inventory where all items are stored in an array, and each page contains a fixed number of items. Each time the player flips an inventory page, the array is sliced to show the correct items.
Note that when slicing, by default, objects are copied by reference, which means that modifying elements in one array changes the other one as well. If this is not what you need, you can set the deep
parameter to true
. This will ensure all elements are copied by value and are actually duplicated. You do this by using the slice(begin, end, step, deep)
method.
var inventory : Array[String] = ["Sword", "Shield", "Helmet", "Boots"]
var slicedInventory : Array[String] = inventory.slice(0, 2)
print(slicedInventory) # Result: ["Sword", "Shield"]
How to Define and Access a 2D Array in Godot?
Godot allows you to define a 2-dimensional array in your code. I have a lot of experience with 2D arrays in C++, and I can tell you that there is almost no case where you need them. They just unnecessarily complicate things. But if your heart is set on it, let’s see how you can implement one in your code, and later on I will mention why I think you shouldn’t use them.
How to Define a 2D Array in Godot?
The definition of a 2-dimensional array in Godot is almost the same as the 1-dimensional array, but with a small addition. You have to set the type of the first array to be Array
.
var array2D : Array[Array] = []
How to Access a 2D Array in Godot?
Again, accessing a 2-dimensional array is pretty similar to accessing a 1-dimensional array, except you need to use two extraction operators [][]
.
var array2D : Array[Array] = [["Sword", "Shield"], ["Helmet", "Boots"]]
var shieldName : String = array2D[0][1]
print(shieldName) # Result: Shield
Why You Should Not Use a 2D Array in Godot
Let me tell you a little secret: you can implement any 2D array logic in a 1D array, and it will work faster.
A 2-dimensional array will always have worse performance since under the hood, the Godot engine has to create nested objects to keep track of the second dimension, which essentially means the path to access an element is longer than in a 1D array. And while the degradation in performance is not significant, it is something you need to be aware of if you are working with large 2D arrays.
Another downside is that you often mix the X and Y coordinates. Let’s be real for a second. The reason why developers rush to use 2D arrays is because they are trying to implement a grid-based game, and a grid by definition is a 2-dimensional entity. So, is the first parenthesis the X or Y coordinate? Is it the row or the column of the board? This little mix-up can be incredibly frustrating down the road.
And finally, it just makes the code messier, as it fills the code with too many parenthesis symbols. So what can you do? How will you survive without a 2D array?
How to Convert a 2D Array to a 1D Array?
Simple – you play with the element indices. All you have to do is ‘flatten’ the 2D array onto one dimension. Now you have a flat list of elements, which represent the entire grid. So how do you access an element? By calculating the sequential index of the required element:
Row * ColumnCount + Column
.
Let’s take an example. Assuming my inventory is a 7 by 7 grid. I want to access my inventory at: row 3, column 5, so what would be the index in a flat 1D array? Index = 3 * 7 + 5 = 26. The requested item will be at index 26 out of the total 49 cells in the grid.
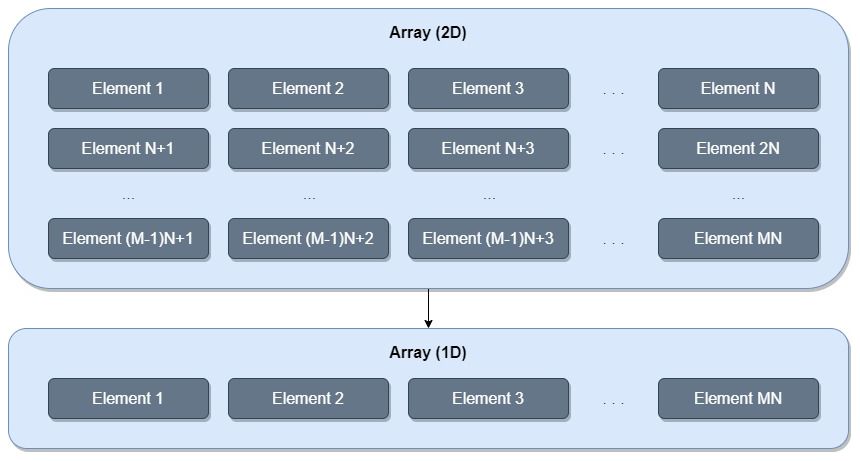
How to Map the Values in an Array?
Mapping is an advanced function of arrays in Godot. Using the map
method, you can transform each value in the array according to a given function with a single line of code. For example, if you want to multiply all existing values by 3.
var anArray : Array[int] = [1, 2, 4, 8]
var mappedArray : Array = anArray.map(Mult3)
print(mappedArray) # Result: [3, 6, 12, 24]
func Mult3(element) -> int:
return element * 3
How to Sort an Array With a Custom Function?
In many cases, you would want to sort an array according to certain custom criteria. Let’s assume you have a list of items a player has collected and now holds in his inventory. How would you go about sorting the items and displaying them based on their monetary value (like when selling items in a market)?
The sorting algorithm uses a comparison function that compares pairs of values in the array. To sort complex objects, you need to implement a function that tells the algorithm how to compare each pair of values. You sort the items by calling sort_custom(callable)
on the arget array.
Assuming you implemented an ‘Item’ node, which contains a Value
property, your comparison function should look something like the code below.
var anArray : Array[InventoryItem] = [itemSword, itemShield, itemHelmet]
anArray.sort_custom(CompareInventoryItem)
func CompareInventoryItem(item1, item2):
return item1.Value < item2.Value
How to Filter an Array Using Custom Criteria?
Filtering an array removes certain elements from it according to a given filter function. The filtering function must return a boolean value (true or false). The filtering algorithm applies the given function to each element and removes the ones that result in a false statement.
var anArray : Array[int] = [6, 2, 4, 8]
var filteredArray : Array[int] = anArray.filter(FilterLowValueItems)
print(filteredArray) # Result: [6, 8]
func FilterLowValueItems(item):
return item >= 6
How to Check a Condition for All or Any Elements in an Array?
The condition functions all
and any
check if a given condition is true for all or any of the elements in the array, respectively. For example, if you want to check if any of the items in the inventory have a monetary value of 10 coins or more.
var anArray : Array[int] = [6, 2, 4, 8]
var containsHighValueItem : bool = anArray.any(ConditionItemAbove10Coins)
print(containsHighValueItem) # Result: false
func ConditionItemAbove10Coins(item):
return item >= 10
More Examples of Arrays and Complex Data Structures in Godot
Arrays can hold any type of data as a list of elements, including dictionaries, other nodes, and even scene instances. The examples outlined below demonstrate some of the useful things you can do with arrays in Godot.
How to Define an Array of Dictionaries?
Each element in the array can be an independent dictionary, which holds key and value pairs.
var dictArray : Array[Dictionary] = [{}, {}, {}, {}] # Array with 4 dictionaries
dictArray[0]["Player_Health"] = 100 # Add an element to the first dictionary
dictArray[0]["Player_Force"] = 25 # Add an element to the first dictionary
print(dictArray) # Result: [{ "Player_Health": 100, "Player_Force": 25 }, { }, { }, { }]
How to Define an Array of Nodes?
Nodes are no different from any other type of object. You can simply append, assign, or insert the nodes into the list and access them normally.
var nodeArray : Array[Node2D] = []
var nodePlayer : Player = get_node("Player")
nodeArray.append(nodePlayer)
print(nodeArray) # Result: [Player:<CharacterBody2D#36003906750>]
How to Define an Array of Scene Instances?
Instantiated scenes are large complex objects, yet they are still allowed to be placed in arrays. Just like any other type, you can append, assign, or insert the scenes into the array and they will work!
var sceneArray : Array[Node2D] = []
sceneArray.append(preload("res://Items/Shield.tscn").instantiate())
sceneArray.append(preload("res://Items/Boots.tscn").instantiate())
print(sceneArray) # Result: [Shield:<StaticBody2D#36641441338>, Boots:<StaticBody2D#36976985678>]
Learning More Game Development in Godot
Godot is awesome, no doubt about it. Go to the Night Quest Games Blog to learn more about Godot and game development in general.
if the information in this articles wasn’t detailed enough and you need more, go to the official Godot Array documnetation page at https://docs.godotengine.org/en/stable/classes/class_array.html.