Tweens are objects that can modify the values of properties of other objects using a mathematical function over time. Godot allows you to expand this functionality using APIs that can invoke methods with changing parameter values, inserting delays, and registering callback methods. The Tween system lets you control the rate of value change by selecting the end value, transition duration, and an interpolation function. Interpolating the position, rotation, scale, or color values of an object will result in simple object animations.
Tweens are not created through the Godot editor but rather through code. This allows you to implement flexible logic that can depend on other modules in your code or even the player’s behavior. For example, a common use of Tweens is for moving objects in the game world. Sometimes, you might want an object to move through the world in a non-linear fashion or in a cyclical manner. Creating a Tween for those cases is a good option.
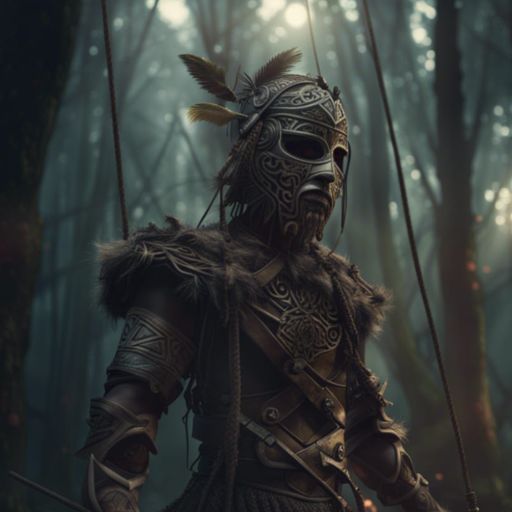
How to Create a New Tween Object in Godot
Godot provides a robust tweening system with various types of Tweeners. In this article, I want to focus on the most common use case of tweens, which is a Property Tweener. A Property Tweener is an object that can modify another object’s property values using a selected mathematical function. There are two ways of creating a tween in Godot, both of which will produce the same result if bound to the same class:
- Calling the
create_tween()
method found in the base Node class creates a Tween object and binds it to the class it’s called from. The method returns a Tween object, which you can then use to define the desired behavior. - Creating a Global Tween object from the main scene tree and binding it to a class can be achieved by executing the following line of code:
get_tree().create_tween().bind_node(object)
.
extends Node2D
func _ready() -> void:
# Create a new bound Tween object
var tween : Tween = create_tween()
extends Node2D
func _ready() -> void:
# Create a new global Tween object
var tween : Tween = get_tree().create_tween().bind_node(self)
Personally, I always use the first method since it’s shorter and achieves the same result.
Modifying Object Properties With Property Tweeners
Now that you know how to create a Tween object, let’s discuss how to actually change properties over time. The main method you should use for this functionality is the tween_property(object, property, final_val, duration)
method. Calling it will interpolate the value of the requested property from its current value to the given final value, with the duration in seconds. The interpolation is performed on the object passed as the first parameter.
- object: The object that contains the target property you want to change over time.
- property: The name of the property you want to change. This value is the actual property name, entered as a string. If you want to interpolate an internal property and are unsure about the property name, you can go to the Inspector panel and hover over the target property with the mouse. You should see a pop-up window that specifies the name of the property, as defined in the code.
- final_val: The final value you want the property to end on.
- duration: The duration (in seconds) of the transition from the current value to the specified final value. A lower duration means the property will reach the final value faster, while a higher duration means it will take longer to reach the final value.
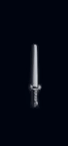
Note: you don’t need to start the tween after calling the tween_property()
method; it will automatically initiate the interpolation in the next frame. However, there are flow control methods you can use to pause, stop, and play the tween, but more on that later.
This method, while very useful, does not tell the whole story of the Tween object. There is some extra functionality provided by Godot to help you control the transition more accurately. Here are some APIs that will enable you to do just that:
as_relative()
is a method that gives you the ability to specify a relative value, instead of a final value. For example, while moving an object, you can specify that the object should move a certain amount of pixels in the desired direction, relative to the current position. The relative value is passed in the final_val parameter.set_trans(transition_type)
sets the transition function of the tween. The transition function is a mathematical function that determines the current value, based on the time left from the specified duration. The transition types are specified below.from(value)
overwrites the current value of the property when the tween goes into effect.set_delay(delay)
sets a time delay (in seconds) between the tween creation and the actual interpolation start.set_ease(ease)
sets the transition ease. This is a further customization of the interpolation function. There are a few ease functions, which I specified below.
Warning: Tweening several properties in the same or in different objects at the same time does not guarantee they all change at the same rate, nor do they complete the tween at the same time.
func MovementAnimation() -> void:
# Create a new Tween object
var movementAnimation : Tween = create_tween()
# Define all parameter for the tween
var object : Node = self # The target node
var property : String = "position" # The target property
var final_val : Vector2 = Vector2.RIGHT * 16 # Final value of the property
var duration : float = 1.6 # Duration (seconds) of the interpolation
# Move the current object 16 pixels to the right (X-axis), stretching over the next 1.6 seconds
# Movement is relative to the objects current position
# Movement uses a linear transition function
movementAnimation.tween_property(object, property, final_val, duration).as_relative().set_trans(Tween.TRANS_LINEAR)
Tween Transition Types in Godot
When using the set_trans()
method, you want to select the appropriate mathematical function for interpolation. There are several options to choose from, so you should pick the one that suits you best. Here are the function descriptions as they appear in the Godot documentation:
- TRANS_LINEAR: The animation is interpolated linearly.
- TRANS_SINE: The animation is interpolated using a sine function.
- TRANS_QUINT: The animation is interpolated with a quintic (to the power of 5) function.
- TRANS_QUART: The animation is interpolated with a quartic (to the power of 4) function.
- TRANS_QUAD: The animation is interpolated with a quadratic (to the power of 2) function.
- TRANS_EXPO: The animation is interpolated with an exponential (to the power of x) function.
- TRANS_ELASTIC: The animation is interpolated with elasticity, wiggling around the edges.
- TRANS_CUBIC: The animation is interpolated with a cubic (to the power of 3) function.
- TRANS_CIRC: The animation is interpolated with a function using square roots.
- TRANS_BOUNCE: The animation is interpolated by bouncing at the end.
- TRANS_BACK: The animation is interpolated backing out at ends.
- TRANS_SPRING: The animation is interpolated like a spring towards the end.
Tween Ease Types in Godot
When using the set_ease(ease)
method, you have a few options to choose from. Here are the tween easing types, as described by the Godot documentation pages:
- EASE_IN: The interpolation starts slowly and speeds up towards the end.
- EASE_OUT: The interpolation starts quickly and slows down towards the end.
- EASE_IN_OUT: A combination of EASE_IN and EASE_OUT. The interpolation is slowest at both ends.
- EASE_OUT_IN: A combination of EASE_IN and EASE_OUT. The interpolation is fastest at both ends.
Animating Objects With Property Tweeners
You can use tweens in Godot to create simple animations using GDScript. Every node2D and node3D has a transform, which includes a position in space, the object’s rotation, and its scale. You can modify these properties to achieve simple animations and visual effects. Additionally, you can play with the alpha channel of the object’s color to achieve transparency effects. Here are some examples of simple effects:
- Interpolate the ‘scale’ vector of the object to make it appear or disappear. Another option is to change the alpha channel of the object from 0.0 to 1.0 to make it appear, or from 1.0 down to 0.0 to make it disappear.
- Rotating an object by interpolating its ‘rotation’ property. This can be done in loops or with different speeds.
- Custom object movement in space can be achieved by interpolating the ‘position’ vector of the object. This is a nice use case for experimenting with transition types.
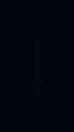
You can also combine tweening of these properties to get more complex effects.
Customizing Property Tweeners Using Advanced Tweening Techniques
In addition to all the customization methods we have already discussed, Godot provides a few more supporting methods, such as tween execution flow control and checking the tween status.
By default, Tween objects execute actions in a chain. This means that calling the tween_property()
method several times on the same Tween object will cause the tween to execute all actions sequentially, in the given order. But in some cases, you might want to execute several interpolations in parallel. To do that, you have two methods:
parallel()
: Makes the current interpolation run in parallel to the previous one. This method should be used if you have a mix of parallel and sequential actions done on the same tween.set_parallel()
: Switches the execution type to be parallel. All tweening actions executed on this Tween object will be done in a parallel manner. It should be used if you have only interpolations that are done in parallel.
What about cases where you want to perform the same interpolations several times in a loop, or even indefinitely until the tween is stopped? There is a method for that too:
set_loops(loops)
: Sets the number of times the tween will execute. Calling this method with a value of zero will make it run forever.
func MovementRotationAnimation() -> void:
# Create a new Tween object
var movementRotationAnimation : Tween = create_tween()
movementRotationAnimation.set_parallel(true) # Set all tweeners to execute in parallel
movementRotationAnimation.set_loops(0) # Set the tween to execute indefinitely
# Move the current object 16 pixels to the right (X-axis) every 1.6 seconds
# Movement is relative to the objects current position
# Movement uses a linear transition function
movementRotationAnimation.tween_property(self, "position", Vector2.RIGHT * 16, 1.6).as_relative().set_trans(Tween.TRANS_LINEAR)
# Rotate the current object 45 degrees every 1 second
# Rotation is relative to the objects current rotation value
# Rotation uses a linear transition function
movementRotationAnimation.tween_property(self, "rotation_degrees", 45, 1).as_relative().set_trans(Tween.TRANS_LINEAR)
How can you start or stop a tween, you ask? Godot has those functions too:
play()
: Resumes a paused or stopped tween.pause()
: Pauses a tween during execution. Retains the current value of the target property.stop()
: Stops the tween during execution. Resets the value of the target property.is_running()
: Returns true if the tween is currently in execution (not paused, stopped, or finished).
My Take on Tweens in Godot
I think many developers don’t fully grasp the power of Tweens in Godot and fail to use them when they should. I hope this article has provided you with the basic knowledge of what tweens are and what you can achieve with them. Godot offers documentation on tweens and their usage in the official documentation page at https://docs.godotengine.org/en/stable/classes/class_tween.html.
I have many more articles about Godot and game development that can enhance your development skills. All you need to do is visit the Night Quest Games Blog and explore the topics you want to learn more about. See you there!
If the information in this article was helpful to you, please consider supporting this blog through a donation. Your contributions are greatly appreciated and allow me to continue maintaining and developing this blog. Thank you!